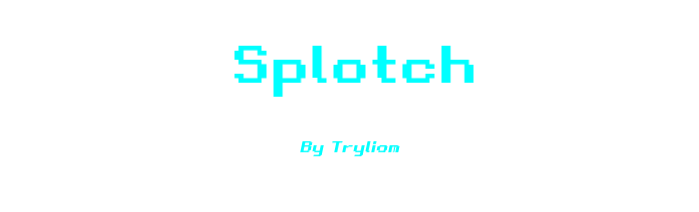
Rollback Game Technical Documentation
Game SAE Network C++ Doc
Building the Game
There are three different executables in the game: the server, the client, and the split screen.
Visual Studio
Open the CMakeLists.txt file with CMake and build the project.
CLion
First, you need to install the following dependencies:
- CMake
- VCPKG
- WSL2 (Windows Subsystem for Linux) with Ubuntu 22.04
WSL2
First, you need to install WSL2. You can follow the instructions on the official documentation.
Second, you need to install Ubuntu 22.04. You can follow the instructions on the official documentation.
Remember to update your system with the following commands:
sudo apt update
sudo apt upgrade
And install the following dependencies
VCPKG
You need to install vcpkg and run the following command:
vcpkg integrate install
SFML dependencies
sudo apt-get install libx11-dev libxrandr-dev libxi-dev libudev-dev libgl1-mesa-dev
During this installation, you can encounter the following error:
libcuda.so.1 is not a symbolic link on WSL2
If this happens, open powershell or cmder as admin and run cmd
or cmd in admin mod
cd \Windows\System32\lxss\lib
del libcuda.so
del libcuda.so.1
Work on cmder but not powershell
mklink libcuda.so libcuda.so.1.1
mklink libcuda.so.1 libcuda.so.1.1
Then, run again the following command:
sudo apt-get install libx11-dev libxrandr-dev libxi-dev libudev-dev libgl1-mesa-dev
CLion
You need to install CLion. You can follow the instructions on the official documentation.
You need to create cmake profile with the following settings.
Debug-windows
Fill the CMAKE options with the following values:
-DCMAKE_TOOLCHAIN_FILE=<Link to your vcpkg.cmake> -DPORT:INT=43845 -DHOST_NAME:STRING="localhost"
The PORT
and HOST_NAME
are the default values. You can change them if you want, but you need to change the debug-server values too.
Example:
-DCMAKE_TOOLCHAIN_FILE=C:\tools\vcpkg\scripts\buildsystems\vcpkg.cmake -DPORT:INT=43845 -DHOST_NAME:STRING="localhost"
Release-windows
Fill the CMAKE options with the following values:
-DCMAKE_TOOLCHAIN_FILE=<Link to your vcpkg.cmake> -DPORT:INT=43845 -DHOST_NAME:STRING="<Your server IP>"
Release-linux
Fill the CMAKE options with the following values:
-DCMAKE_TOOLCHAIN_FILE=<Link to your vcpkg.cmake in WSL> -DPORT:INT=43845
Example:
-DCMAKE_TOOLCHAIN_FILE=~/dev/vcpkg/scripts/buildsystems/vcpkg.cmake -DPORT:INT=43845
You need to run each profile for them to recognize HOST_NAME and PORT.
Server
The server is responsible for managing the game state and sending it to the clients. It is implemented using SFML. The server runs the game loop and the input system.
Client
The client is responsible for rendering the game state and sending the player’s inputs to the server. It is implemented using SFML. The client runs the game loop and the input system.
Split Screen
The split screen implements two clients on the same screen and the server. It is implemented using SFML. The split screen runs the game loop and the input system.
Network
The network is implemented using SFML. The network model is based on the client-server model. The network protocol is used in TCP and UDP.
Packet Structure
The packet structure is composed of a packet type and methods to serialize and deserialize the packet that needs to be implemented by the derived classes.
Add a new Packet
To add a new packet, you need to create a new class that inherits from the Packet
class.
In the derived classes, you need to add attributes that you want to send and implement the Write
and Read
methods.
Per example, the UDPAcknowledgePacket
packet is used to acknowledge the reception of a packet.
You need to create a new packet type using your own enum starting from the last value of the PacketType
enum.
At the start of the program, you need to register your packets using the PacketManager
namespace.
They need to be in the same order as the MyPacketType
enum.
Rollback
Rollback is a technique done by rewinding the game state to a previous point in time and replaying the game from that point if the game state is inconsistent between both players.
The game state is stored in GameData
class, so all additional gameplay should be stored in this class.
The class is derived for client and server to manage who is the current player and for a client to manage the player animations to become consistent on rollback.